
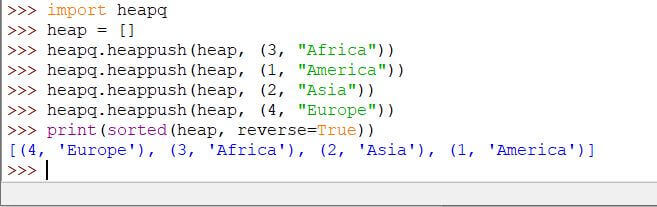
The implementation uses a min-heap, meaning that elements with lower priority values are popped first.
#Python priority queue update value how to
The example provided demonstrates how to use the `heapq` module from the Python Standard Library to easily implement a priority queue. If you need a max-heap (where elements with higher priority values are popped first), you can simply negate the priority values when pushing items to the queue. Please note that this implementation uses a min-heap, meaning that elements with lower priority values are popped first. Coming from Java, I am trying to implement A algorithm in python and Im having trouble sorting vertices in my graph that have equal f scores. It inserts elements in descending order, and gives the maximum value the highest. The `push` and `pop` methods insert and remove items from the priority queue, respectively. The following code in python implements a priority queue using a binary heap. In this example, we create a `PriorityQueue` class that wraps the functionality provided by the `heapq` module. Print(pq.peek()) # Output: task4, because it hasn't been popped yet Raise Exception("Priority queue is empty") Heapq.heappush(self.queue, (priority, item)) Here’s an example of how you can implement a priority queue:
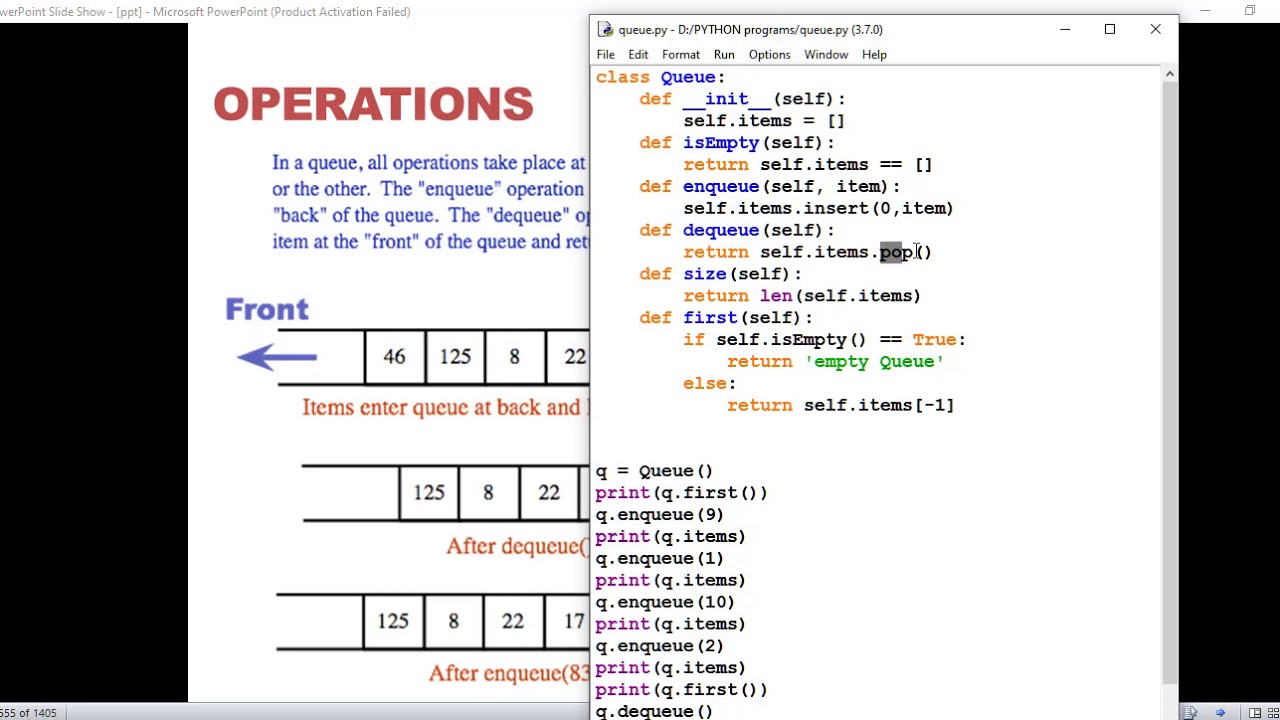
In some implementations, if two elements have the same priority. The `heapq` module provides functions for creating min-heaps using lists. In a priority queue, elements with high priority are served before elements with low priority. In Python, you can easily implement a priority queue using the `heapq` module from the Python Standard Library.
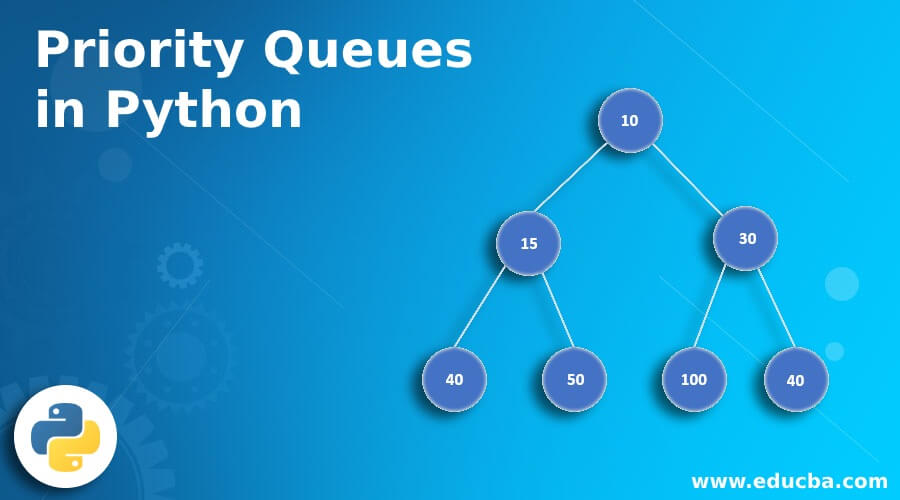
Please note that this implementation uses a min-heap, meaning that elements with lower priority values are popped first if you need a max-heap (where elements with higher priority values are popped first), you can simply negate the priority values when pushing items to the queue. We create a `PriorityQueue` class that wraps the functionality provided by this module, and demonstrate its usage with some examples. This blog post provides an example of how to implement a priority queue in Python using the `heapq` module from the Python Standard Library.
